questions i got wrong on all of my unity quizzes
Question 5
If you want to move the character up continuously as the player presses the up arrow, what code would be best in the two blanks below:
GetKey(KeyCode.UpArrow)
GetKeyDown(UpArrow)
GetKeyUp(KeyCode.Up)
GetKeyHeld(Vector3.Up)
Incorrect
“Input.GetKey” tests for the user holding down a key (as opposed to KeyKeyDown, which test for a single press down of a Key).
Question 10
You’re trying to create some logic that will tell the user to speed up if they’re going too slow or to slow down if they’re going too fast. How should you arrange the lines of code below to accomplish that?
4, 6, 1, 2, 5, 9, 7, 8, 3
void Update() { if (speed < 10) {Debug.Log(speedUp); } else if (speed > 60) { Debug.Log(slowDown); } } private float speed; private string slowDown = "Slow down!"; private string speedUp = "Speed up!";
6, 1, 2, 5, 7, 8, 3, 4, 9
if (speed < 10) { Debug.Log(speedUp); } else if (speed > 60) { Debug.Log(slowDown); } private float speed; private string slowDown = "Slow down!"; private string speedUp = "Speed up!"; void Update() { }
7, 8, 3, 4, 6, 5, 2, 1, 9
private float speed; private string slowDown = "Slow down!"; private string speedUp = "Speed up!"; void Update() { if (speed < 10) { Debug.Log(slowDown); } else if (speed > 60) { Debug.Log(speedUp); } }
7, 8, 3, 4, 6, 1, 2, 5, 9
private float speed; private string slowDown = "Slow down!"; private string speedUp = "Speed up!"; void Update() { if (speed < 10) { Debug.Log(speedUp); } else if (speed > 60) { Debug.Log(slowDown); } }
Incorrect
All variables should be declared first, then the void method, then the if-condition telling them to speed up, then the else condition telling them to slow down.
Question 6
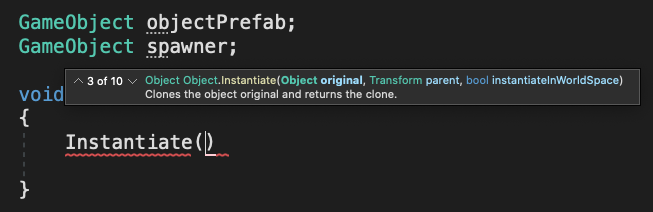
No Comments