2nd quiz
Question 1
If it says, “Hello there!” in the console, what was the code used to create that message?
Debug.Log() prints messages to the console and can accept String parameters between quotation marks, such as “Hello there!”
If you want to destroy an object when its health reaches 0, what code would be best in the blank below?
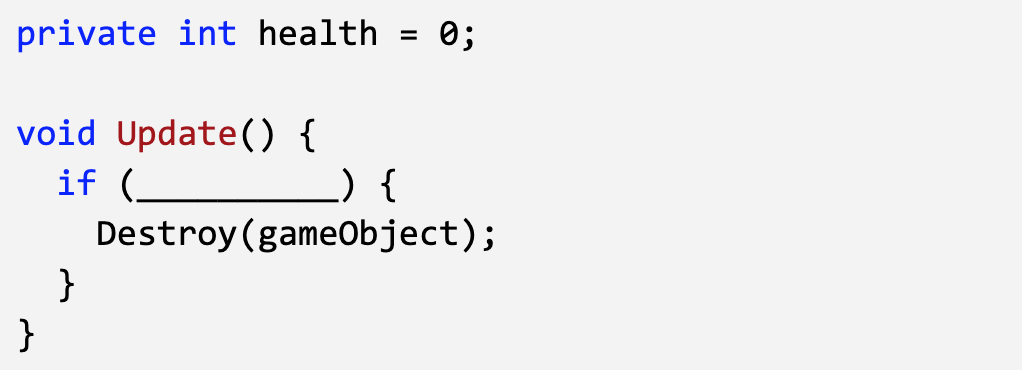
Since the “health” variable is an int, anything less than 1 would be “0”. The sign for “less than” is “<”.
The code below creates an error that says, “error CS1503: Argument 1: cannot convert from 'UnityEngine.GameObject[]' to 'UnityEngine.Object'”. What could you do to remove the errors?
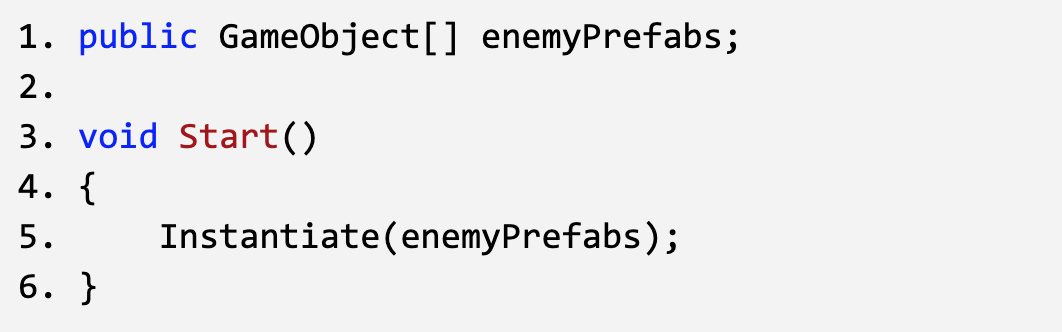
“GameObject[]” is a GameObject array. You cannot instantiate an array, but you
can instantiate an object inside an array. So you could either remove the array and have Instantiate use an individual object (option A) or you could use an GameObject index of that Array (option D), but both would not work.Which comment best describes the following code?
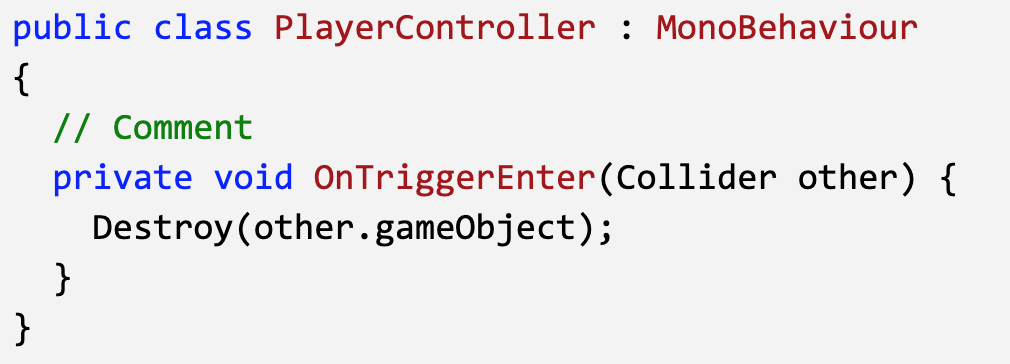
Since it’s inside the PlayerController class, and it is destroying
other.gameObject, it is destroying something that the player collides with.If you want to move the character up continuously as the player presses the up arrow, what code would be best in the two blanks below:
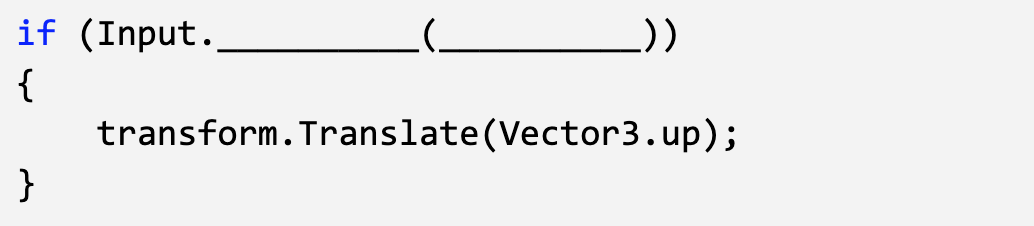
“Input.GetKey” tests for the user holding down a key (as opposed to KeyKeyDown, which test for a single press down of a Key).
Read the documentation from the Unity Scripting API and the code below. Which of the following are possible values for the randomFloat and randomInt variables?
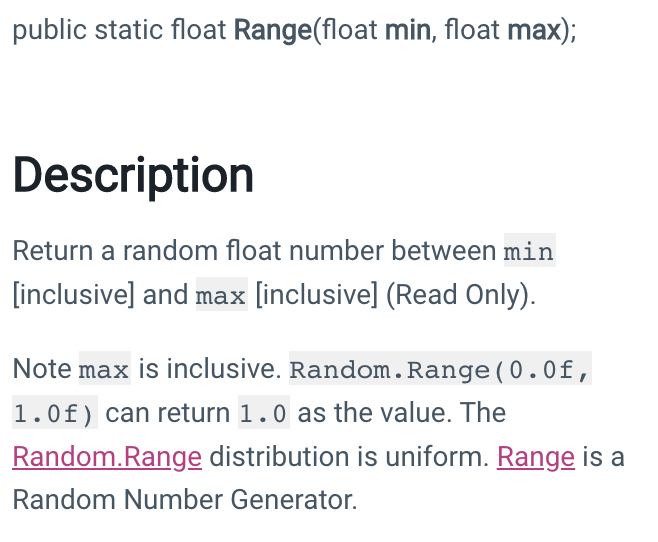
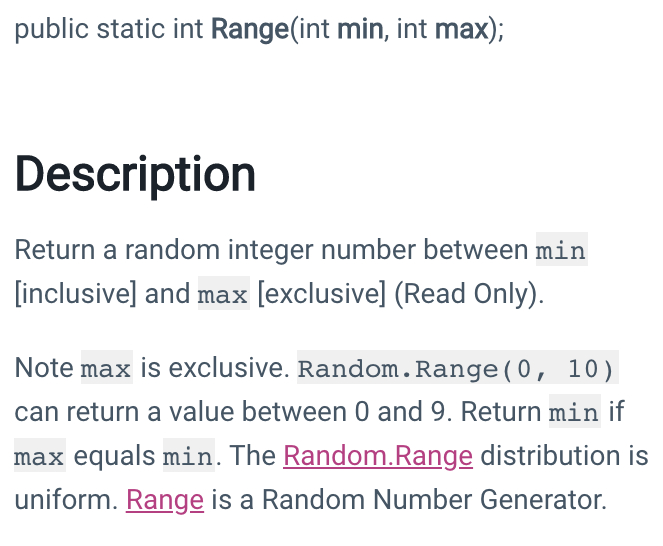

As it says in the documentation, Random.Range does
not include the maximum value for integers, but does include the maximum value for floats. This means that randomInt cannot be 100, but randomFloat can be.You are trying to randomly spawn objects from an array. However, when your game is running, you see in the console that there was an “error at Assets/Scripts/SpawnManager.cs:5. IndexOutOfRangeException: Index was outside the bounds of the array.” Which line of code should you edit in order to resolve this problem and still retain the random object functionality?
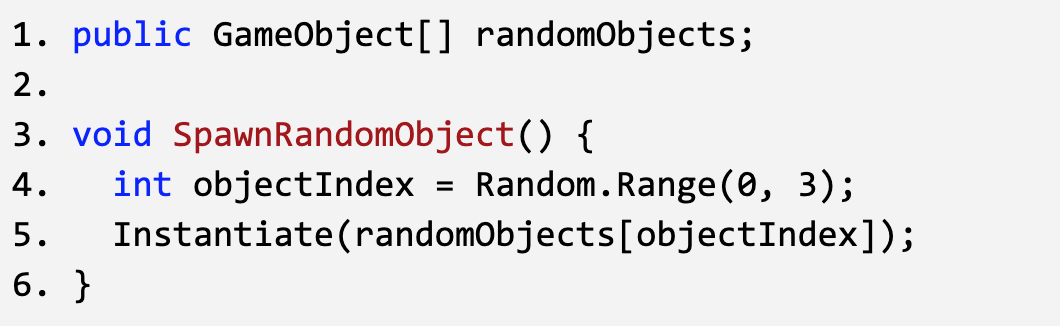
Line 4, which generates the objectIndex, must be generating an index value that is too high for the number of objects in the array. The best thing to do would be to change it to “Random.Range(0, randomObjects.Length);
If you have made changes to a prefab in the scene and you want to apply those changes to all prefabs, what should you click?
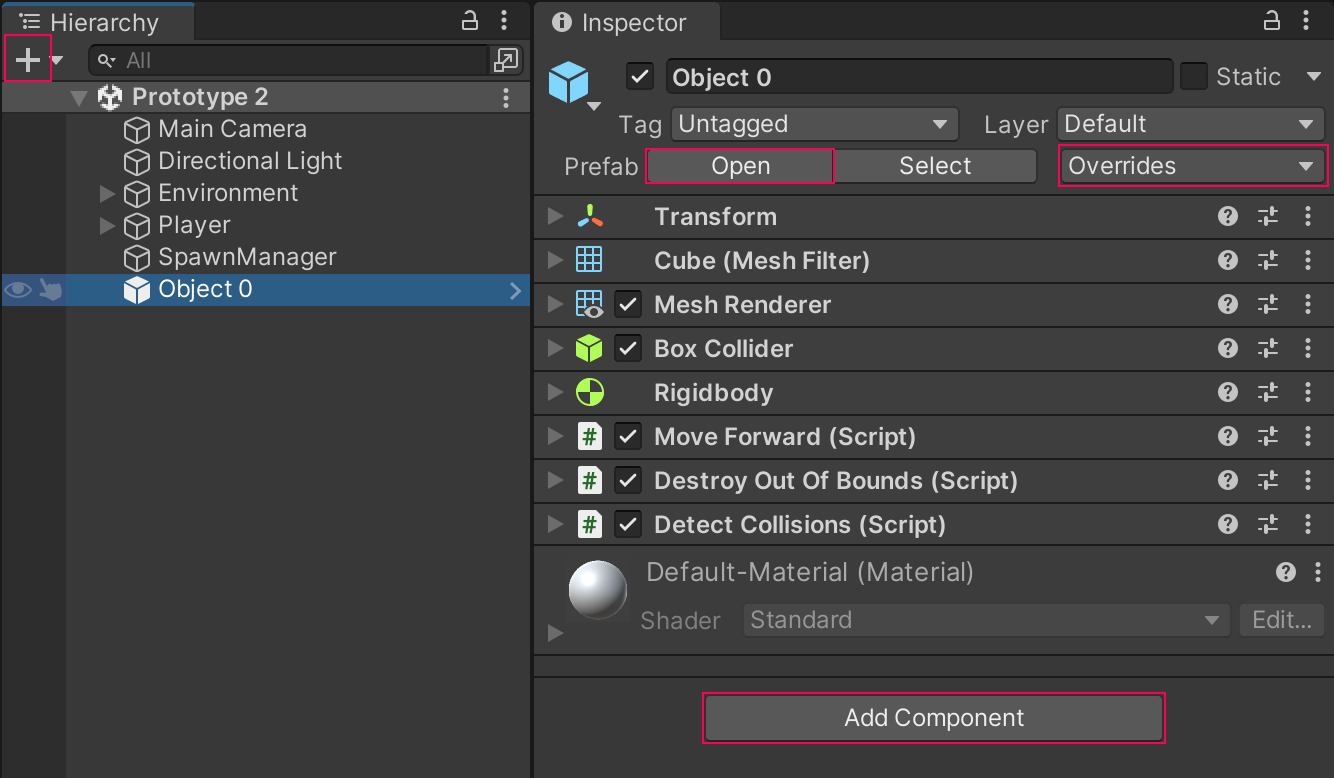
The “Override” drop-down will allow you to apply any changes you’ve made to your individual prefab to the original prefab object.
Read the documentation from the Unity Scripting API below. Which of the following is a correct use of the InvokeRepeating method?
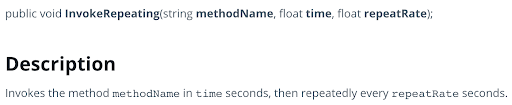
According to the Scripting API, InvokeRepeating requires a string parameter, then two floats.
You’re trying to create some logic that will tell the user to speed up if they’re going too slow or to slow down if they’re going too fast. How should you arrange the lines of code below to accomplish that?
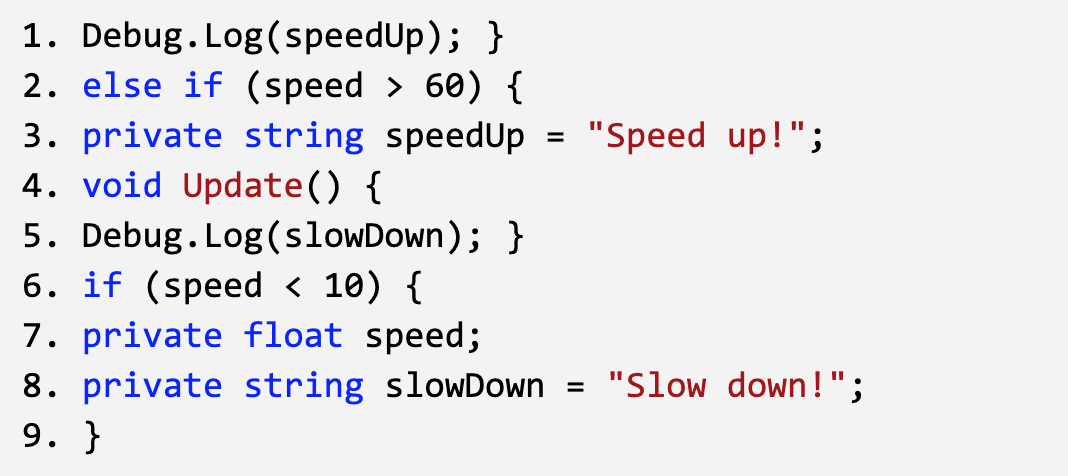
All variables should be declared first, then the void method, then the if-condition telling them to speed up, then the else condition telling them to slow down.